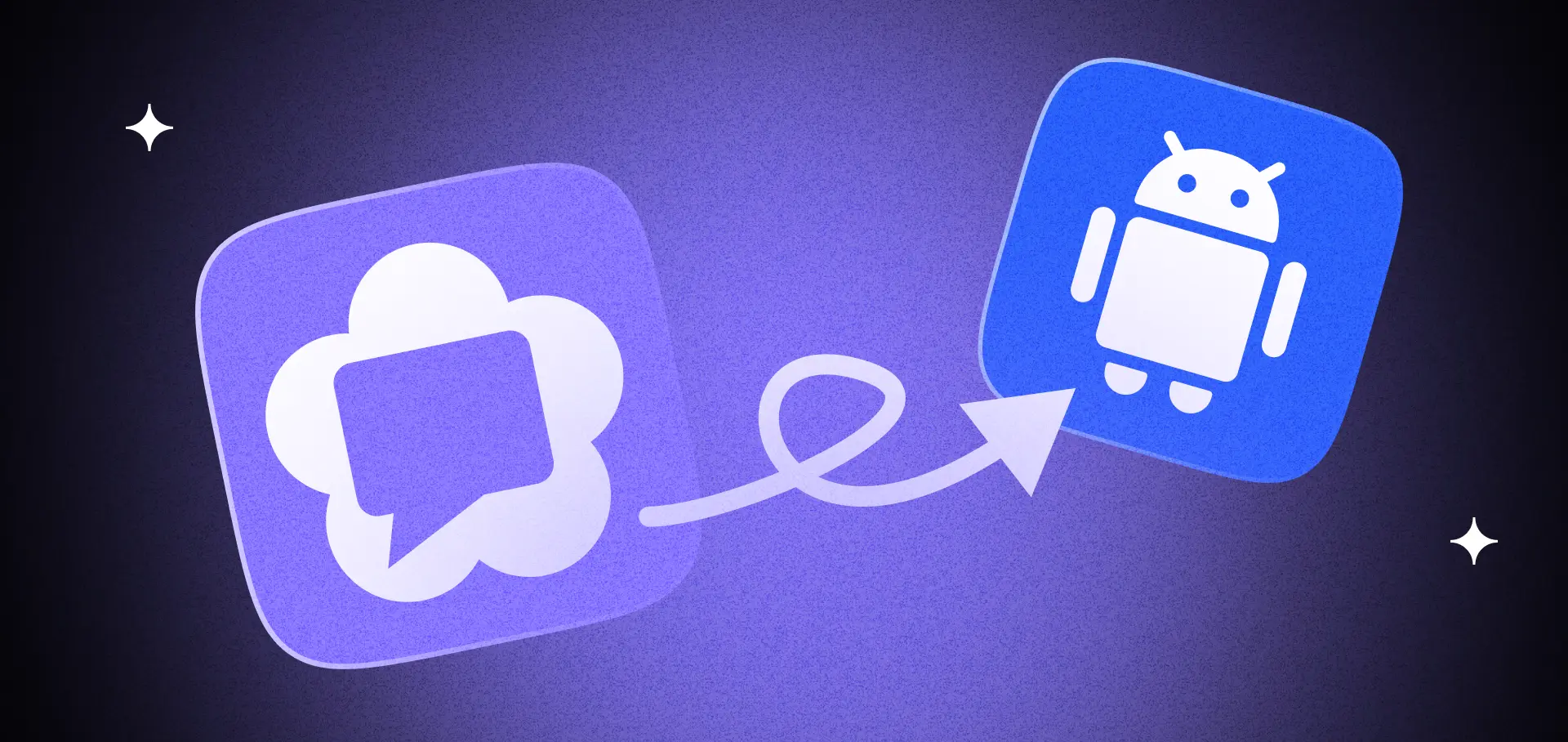
Adding screen sharing to your Android app using WebRTC opens up amazing possibilities for live video and audio sharing. This popular technology lets your users share their screens in real-time, making collaboration and communication much easier. The process combines Android Studio with the WebRTC library, along with some smart background handling to keep everything running smoothly. While it might sound complex, breaking it down into simple steps makes implementation straightforward. From setting up basic permissions to adding user-friendly notifications, you'll learn how to create a reliable screen sharing feature that your users will love. Let's walk through how to build this into your Android app, including some practical tips for better performance and security.
Key Takeaways
- Use the MediaProjection API to capture and share the device’s screen content.
- Request necessary permissions for screen capture and accessibility.
- Integrate WebRTC Library for real-time communication and streaming.
- Set up persistent background service for uninterrupted streaming.
- Ensure efficient resource management to optimize battery usage and performance.
Understanding WebRTC Screen Sharing Technology
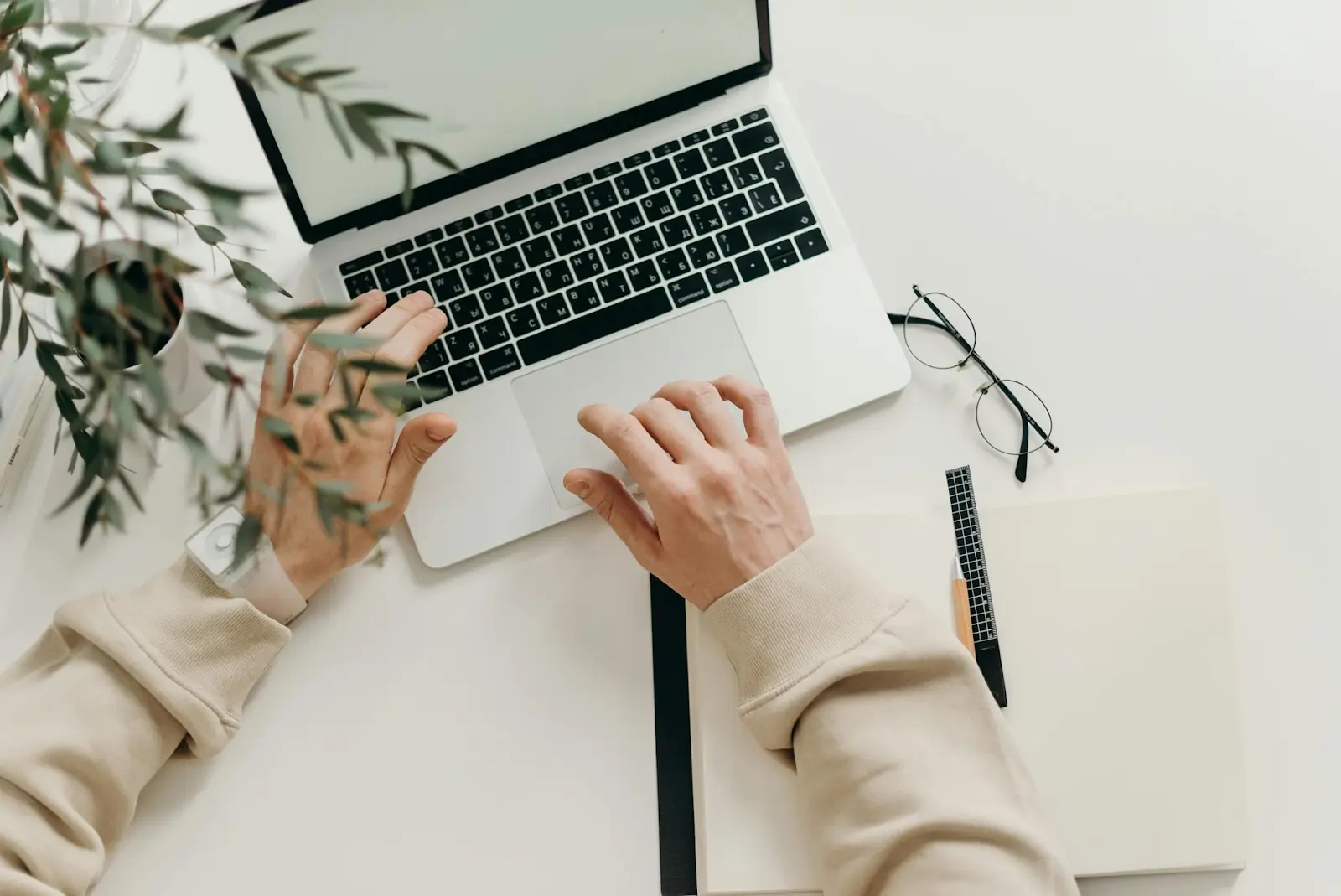
WebRTC screen sharing allows users to display their screen to others in real-time. Studies have shown that WebRTC provides more reliable and higher-quality video and audio synchronization compared to traditional video conferencing solutions, leading to improved user satisfaction (Patel et al., 2021).
For Android development, WebRTC offers core benefits like low latency and peer-to-peer connections, which make screen sharing smooth and fast. Before starting, developers need to know the technical requirements, such as having Android 5.0 or later and ensuring the app has the right permissions to capture and share the screen.
Why Trust Our WebRTC Implementation Expertise?
At Fora Soft, we've been at the forefront of multimedia development for over 19 years, specializing in WebRTC implementations across various platforms. Our team has successfully delivered numerous video streaming solutions, maintaining a remarkable 100% project success rating on Upwork. This perfect score reflects our deep understanding of WebRTC technology and its practical applications in real-world scenarios.
We've implemented WebRTC solutions for diverse industries, including video surveillance, e-learning, and telemedicine platforms. Our expertise extends beyond basic implementation – we've enhanced WebRTC capabilities with AI-powered features for bandwidth optimization and video quality improvement. This combination of WebRTC proficiency and AI integration allows us to deliver solutions that not only work flawlessly but also adapt intelligently to varying network conditions and user requirements.
🎯 Ready to leverage our WebRTC expertise? Let's discuss your project! Schedule a free consultation – we promise no pushy sales talk, just honest tech discussion.
Core Benefits of WebRTC for Android Development
Enhance the capabilities of Android applications with the integration of WebRTC, a potent technology that enables real-time communication.
WebRTC allows developers to add real-time communication capabilities, such as voice and video calling, directly within an app. One of the core benefits is the ability to establish a peer connection, which means users can communicate directly without needing an intermediary server. This peer-to-peer architecture significantly reduces latency during real-time communications, making it ideal for time-sensitive applications (Bacco et al., 2018).
WebRTC is also highly flexible, working well with various network conditions and devices. The technology processes communications at the user level, which significantly reduces processing delays and connection times while adapting transmission rates to available bandwidth (Ha et al., 2020). Moreover, its open-source nature means it's free to use and has a strong community support for continuous improvement and security updates. The technology supports data channels, too, enabling file sharing and more during a live session.
Technical Requirements and Prerequisites

To implement WebRTC screen sharing on Android, developers need to understand specific technical requirements.
This includes particular Android permissions, such as those for accessing the screen and microphone.
Moreover, setting up the correct development environment is vital for integrating WebRTC features effectively.
Required Android Permissions
Although WebRTC screen sharing allows the transmission of desktop or application screens in real-time, enabling this feature on Android devices requires specific permissions.
Primarily, apps need to have accessibility permission. This enables them to read the screen’s content and capture gestures through accessibility services. It's vital to inform users that these permissions are necessary for screen sharing functionality.
Moreover, permissions for recording audio and video may be required depending on the app's features.
Development Environment Setup
While integrating WebRTC screen sharing into an app, developers must first set up a suitable development environment. This part of the development process guarantees that all the necessary tools and libraries are in place.
For Android devices, specific configurations are required to enable screen sharing successfully. Key components of the setup include:
- Android Studio: The official IDE for Android development, essential for writing and testing code.
- WebRTC Library: The core component for real-time communication, which needs to be integrated into the project.
- Java Development Kit (JDK): Necessary for compiling Java code, a vital step in the development process.
- Gradle Build Tool: Manages dependencies and handles the build process efficiently.
- Screen Capture Permissions: Essential for capturing and sharing the screen on Android devices.
The development environment setup is a critical step that guarantees smooth integration and functionality of WebRTC screen sharing.
Implementing WebRTC Screen Sharing in Android
Implementing WebRTC screen sharing in Android involves setting up the MediaProjection API to capture the screen.
Establishing peer connections is essential for transmitting the screen data between users.
Moreover, there are advanced implementation features that enhance the overall user experience.
Setting Up MediaProjection API
To implement WebRTC screen sharing on Android, developers need to understand the MediaProjection API, which allows apps to capture and share the device's screen.
This process involves requesting user permissions to guarantee privacy and security. Once permissions are granted, the API handles screen capture, enabling real-time sharing of the device's display content.
Requesting User Permissions
Requesting user permissions is an essential step in setting up WebRTC screen sharing on Android using the MediaProjection API.
To ensure seamless functionality and a smooth user experience, Android apps must prompt users to grant several key permissions:
- Foreground Service Permission: This allows the app to run in the background, vital for maintaining a stable screen sharing session.
- Microphone Access: Necessary if the app includes audio alongside screen sharing, enhancing the overall user experience.
- Screen Capture Permission: Enables the app to record and broadcast the device's screen content.
- Display Over Other Apps: Allows the app to show a floating window for easier user interaction.
- System Alert Window Permission: Required for creating overlays that provide users with notifications and controls during screen sharing.
Upon obtaining these user permissions, the app can efficiently employ the MediaProjection API for screen sharing.
Handling Screen Capture
Once user permissions are successfully granted, the next essential step involves handling screen capture using the MediaProjection API.
This API is necessary for capturing screen content in the implementation of screen sharing on Android devices. Developers create a MediaProjection object, which allows the app to capture the device's display.
This object is then used to start the screen capture process, enabling the app to share the screen content over a WebRTC connection. The API also handles stopping the capture when needed, ensuring smooth control over the screen sharing feature.
Establishing Peer Connections
To enable WebRTC screen sharing on Android, developers need to set up ICE servers, which help establish connections between peers.
These servers manage the process of finding the best path for data to travel, ensuring smooth media stream transmission. The media streams, which include the screen content being shared, are then managed by the app to maintain quality and synchronization during the sharing session.
ICE Server Configuration
When setting up WebRTC screen sharing on Android, it's crucial to tackle the configuration of ICE servers. These servers help manage the peer connection process by finding the best path for media streams.
During setup, developers must specify:
- STUN server URLs: These help determine the public IP addresses of the devices.
- TURN server credentials: These include URLs, usernames, and passwords for relaying media when direct connections fail.
- Handling network changes: Implementing listeners to detect and adjust to network alterations.
- Managing disconnections: Setting up mechanisms to reconnect or notify users when the connection is lost.
- Optimizing performance: Fine-tuning settings to guarantee minimal latency and high-quality video during screen sharing.
Managing Media Streams
After configuring ICE servers, the next step in implementing WebRTC screen sharing on Android involves managing media streams.
This means handling both the outgoing screen share stream and any incoming video streams. Developers can set parameters like video resolutions to control the quality and bandwidth usage. They use APIs to attach these streams to a peer connection, allowing users to share their screens and see others' video feeds.
It's vital to manage these streams efficiently to guarantee smooth, real-time communication. For instance, developers can dynamically adjust the video resolution based on the device's capabilities and network conditions, providing an ideal experience for users.
Advanced Implementation Features
Integrating WebRTC screen sharing in Android can be enhanced with background service integration, ensuring the app runs smoothly even when it's not actively used.
AI-powered bandwidth optimization can automatically adjust video quality based on network conditions, making sure users have the best experience possible.
Real-time video enhancement features can improve the clarity and sharpness of the shared screen, providing a better visual experience for all participants.
Background Service Integration
Incorporating WebRTC screen sharing into an Android application can be greatly enhanced by utilizing background service integration.
A Background Service allows the Video Stream to continue even when the app isn't actively being used. Users can switch to other apps or turn off the screen without interrupting the stream.
Key aspects of background service integration include:
- Persistent Streaming: Keeps the video stream alive while the app is in the background.
- Resource Management: Efficiently manages system resources to prevent excessive battery drain.
- Notification Integration: Provides users with real-time updates about the streaming status.
- Seamless User Experience: Makes certain users can multitask without worrying about the stream.
- Error Handling: Automatically manages and recovers from common streaming issues.
AI-Powered Bandwidth Optimization
One of the most considerable challenges in WebRTC screen sharing on Android devices is maintaining a stable stream quality under varying network conditions. Implementing adaptive bitrate streaming can enhance user experiences by dynamically adjusting video quality based on real-time network conditions, particularly in multi-user wireless settings where network interference is common (Gao et al., 2018).
Developers use AI-powered bandwidth optimization to modify video quality based on the speed and stability of internet connections. This helps keep the stream smooth, even when the user's connection is weak or unstable.
AI can predict and accommodate changes in the network, making sure the video doesn't freeze or break up. This technique uses machine learning algorithms to analyze network data and optimize the bandwidth use in real-time.
It's a smart way to make screen sharing more reliable, even on shaky networks.
Real-Time Video Enhancement
For developers looking to enhance the user experience in WebRTC screen sharing on Android, real-time video improvement is an essential feature to explore. This involves dynamically adjusting levels of video quality based on network conditions and device capabilities.
By implementing such enhancements, developers can guarantee that incoming videos are clear and smooth, even under fluctuating network environments.
Key aspects of real-time video enhancement include:
- Adaptive Bitrate: Automatically adjusts the bitrate of the video stream to match the current network speed.
- Frame Rate Control: Dynamically changes the frame rate to maintain smooth playback during network congestion.
- Noise Reduction: Filters out background noise to improve audio clarity, important for screen sharing with voiceovers.
- Low Light Enhancement: Improves video quality in dimly lit environments by adjusting brightness and contrast.
- Error Correction: Detects and corrects errors in the video stream to minimize disruptions and guarantee continuous playback.
Optimizing Performance and Security
To make WebRTC screen sharing better, developers can use techniques that adjust the amount of data sent based on the internet speed. They can also add extra security steps to protect the data being shared.
Testing and finding bugs is also important to guarantee that the screen sharing feature works smoothly.
Smart Bandwidth Management Techniques
WebRTC's smart bandwidth management includes machine learning adjustment to predict and adjust to network changes in real-time. This helps optimize performance by ensuring smooth screen sharing even when bandwidth fluctuates.
Moreover, multi-path streaming support allows the system to send data over multiple paths simultaneously, enhancing security and reliability.
Machine Learning Adaptation
As developers integrate WebRTC for screen sharing, they encounter a critical challenge: maintaining peak performance and security while managing volatile bandwidth conditions.
Machine learning is now a key player in optimizing WebRTC in Android browsers.
Here's how it contributes:
- Machine learning models can predict network conditions and adjust video quality to prevent disruptions.
- These models analyze past data to learn and adapt to different network situations.
- They can also detect and respond to sudden bandwidth changes in real-time.
- Security is enhanced by machine learning, which can spot unusual patterns that might indicate a threat.
- Over time, the system improves, making screen sharing smoother and more secure for users.
Multi-Path Streaming Support
Continuing from machine learning's role, another method developers use to boost WebRTC screen sharing performance is multi-path streaming support.
This technique allows data to travel through multiple connection paths simultaneously, utilizing various ice candidates. If one path fails, data seamlessly switches to another, ensuring uninterrupted streaming. WebRTC applications have demonstrated superior connection reliability across different network scenarios and browsing platforms (Tarim & Tekin, 2019).
This strategy enhances reliability and optimizes bandwidth usage, improving the overall user experience.
Enhanced Security Measures
WebRTC implements end-to-end encryption, ensuring that everything shared, including screen data, is scrambled until it reaches the person on the other end.
Users have full control over their privacy, with the ability to start or stop sharing their screen at any moment.
Developers can include features like permission requests and visible indicators to enhance user awareness of when sharing is active.
End-to-End Encryption Implementation
Implementing end-to-end encryption in software applications enhances both performance and security, making it a critical consideration for developers.
For WebRTC screen sharing on Android, end-to-end encryption secures communication between peers, guaranteeing data integrity and confidentiality.
Key aspects include:
- Data Encryption: Encrypts data before sending, guaranteeing it remains secure during transmission.
- Key Exchange: Securely shares encryption keys between users or devices.
- Tampering Prevention: Detects any modifications to the data during transmission.
- Performance Optimization: Reduces overhead by balancing encryption strength and speed.
- Cross-Platform Compatibility: Guarantees encryption works smoothly across different devices and operating systems.
User Privacy Controls
After securing data through end-to-end encryption, developers must consider how to give users control over their privacy during WebRTC screen sharing.
This includes allowing users to adjust device settings to limit what's shared. For example, users could exclude specific applications or windows from being broadcasted.
Furthermore, developers can implement device policies that automatically pause screen sharing when a user switches to a sensitive app, like a password manager. This approach guarantees that users have fine-grained control over their privacy, making the screen sharing experience more secure and comfortable.
Users can also be given options to quickly enable or disable screen sharing with a single tap, providing them with immediate control over their data broadcast.
Testing and Debugging Strategies
WebRTC screen sharing can encounter performance glitches and security concerns.
Developers use tools like Chrome's Lighthouse and WebRTC Internals to spot issues.
Common problems include high latency and echoes, often fixed by tweaking media constraints and handling ice connection failures.
Performance Monitoring Tools
To ensure reliable and high-performing WebRTC screen sharing, developers rely on performance monitoring tools that provide real-time insights into system behavior.
These tools are crucial for identifying bottlenecks, improving speed, and maintaining security throughout the application. Some of the most effective tools include:
- Callstats.io: This tool monitors the quality of screen share streams, providing detailed reports on latency, jitter, and packet loss.
- Chrome DevTools: Built into Chrome, it helps analyze WebRTC performance, including data channels and media stream quality.
- Janus WebRTC Server: Offers monitoring capabilities to track the performance of WebRTC sessions, including CPU and bandwidth usage.
- TestRTC: Provides end-to-end testing and monitoring for WebRTC applications, helping identify issues in screen share functionality.
- WebRTC Internals: A Chrome extension that offers detailed information on WebRTC statistics, useful for debugging performance issues.
Common Issues and Solutions
Once developers have monitoring tools in place, they often encounter common issues that need addressing.
Issues like a device crash during screen sharing can stem from problems with the signaling server. High latency is usually due to poor network conditions or inefficient coding in the app itself.
Security vulnerabilities might arise if the data sent between users isn't properly encrypted. Ensuring the app handles errors gracefully can prevent sudden crashes.
Testing with various devices and networks helps identify and fix these issues.
WebRTC Implementation Roadmap Navigator
Planning to implement WebRTC screen sharing in your Android app? Use our interactive roadmap navigator to visualize the implementation process, track your progress, and understand the key steps involved. This tool helps product owners and developers plan their WebRTC integration journey more effectively, highlighting critical decision points and requirements at each stage.
Frequently Asked Questions
What Devices Support Webrtc Screen Sharing?
The current question concerns the devices that support WebRTC screen sharing. Modern Android devices (running Android 5.0 or later), iOS devices (running iOS 11 or later), and most desktop browsers, including Chrome, Firefox, and Edge, support WebRTC screen sharing. However, device-specific limitations and browser versions may impact functionality.
Can You Record Screen Sharing Sessions?
Recording screen sharing sessions is possible. Various software and tools can capture the screen content during a session. These tools often allow for saving the recording as a video file for later viewing or sharing.
How Does Screen Sharing Affect Battery Life?
Screen sharing can notably impact battery life due to the continuous encoding and transmission of screen data, increased CPU and GPU usage, and the constant use of the device's screen and network interfaces. This leads to a higher energy consumption, reducing the device's battery lifespan between charges. Moreover, the battery drain is more pronounced with higher screen resolutions and refresh rates, as well as poor network conditions that require more energy for data transmission.
What Are the Common Issues Users Face?
Common issues users face include difficulty initiating the screen share, poor video quality, latency, and compatibility problems with different devices and browsers. Moreover, users may encounter unstable connections, dropped frames, and increased battery drain. Permission issues and security concerns may also arise, as users might be prompted to adjust system settings or grant app permissions to enable screen sharing.
Is There a Way to Customize the Screen Sharing UI?
Customizing the screen sharing UI is possible through various means. In Android, developers can alter the layout and design of the UI components used for screen sharing. This may involve modifying the presentation of the screen sharing button, the selection dialog for choosing the screen to share, and any on-screen controls that appear during the sharing session. Such customization typically requires a good understanding of Android’s UI framework and may involve creating custom views or modifying existing ones.
To Sum Up
WebRTC screen sharing is a potent tool for Android apps, offering real-time communication and collaboration. It requires using the MediaProjection API and optimizing performance for smooth user experience. Enhanced security measures protect data during transmission. Testing and debugging guarantee reliability. Product owners can explore this technology to improve their apps, making them more interactive and user-friendly.
⭐ Ready to take the next step? Our team of WebRTC experts is here to help you implement these features flawlessly. Book a call today and let's bring your vision to life!
References
Bacco, M., Catena, M., Cola, T., Gotta, A., & Tonellotto, N. (2018). Performance analysis of WebRTC-based video streaming over power constrained platforms. In 2018 IEEE Global Communications Conference (GLOBECOM) (pp. 1-7). IEEE. https://doi.org/10.1109/glocom.2018.8647375
Gao, L., Tang, M., & Pang, H., et al. (2018). Multi-user cooperative mobile video streaming: Performance analysis and online mechanism design. arXiv preprint. https://doi.org/10.48550/arxiv.1805.09249
Ha, V., Chai, R., & Nguyen, H. (2020). A telepresence wheelchair with 360-degree vision using WebRTC. Applied Sciences, 10(1), 369. https://doi.org/10.3390/app10010369
Patel, V., Li, C., & Rye, V., et al. (2021). A comparison of WebRTC and conventional videoconferencing for synchronized remote medical image presentation. Journal of Digital Imaging, 35(1), 68-76. https://doi.org/10.1007/s10278-021-00544-0
Tarim, E., & Tekin, H. (2019). Performance evaluation of WebRTC-based online consultation platform. Turkish Journal of Electrical Engineering & Computer Sciences, 27(6), pp.4314-4327. https://doi.org/10.3906/elk-1903-44
Comments