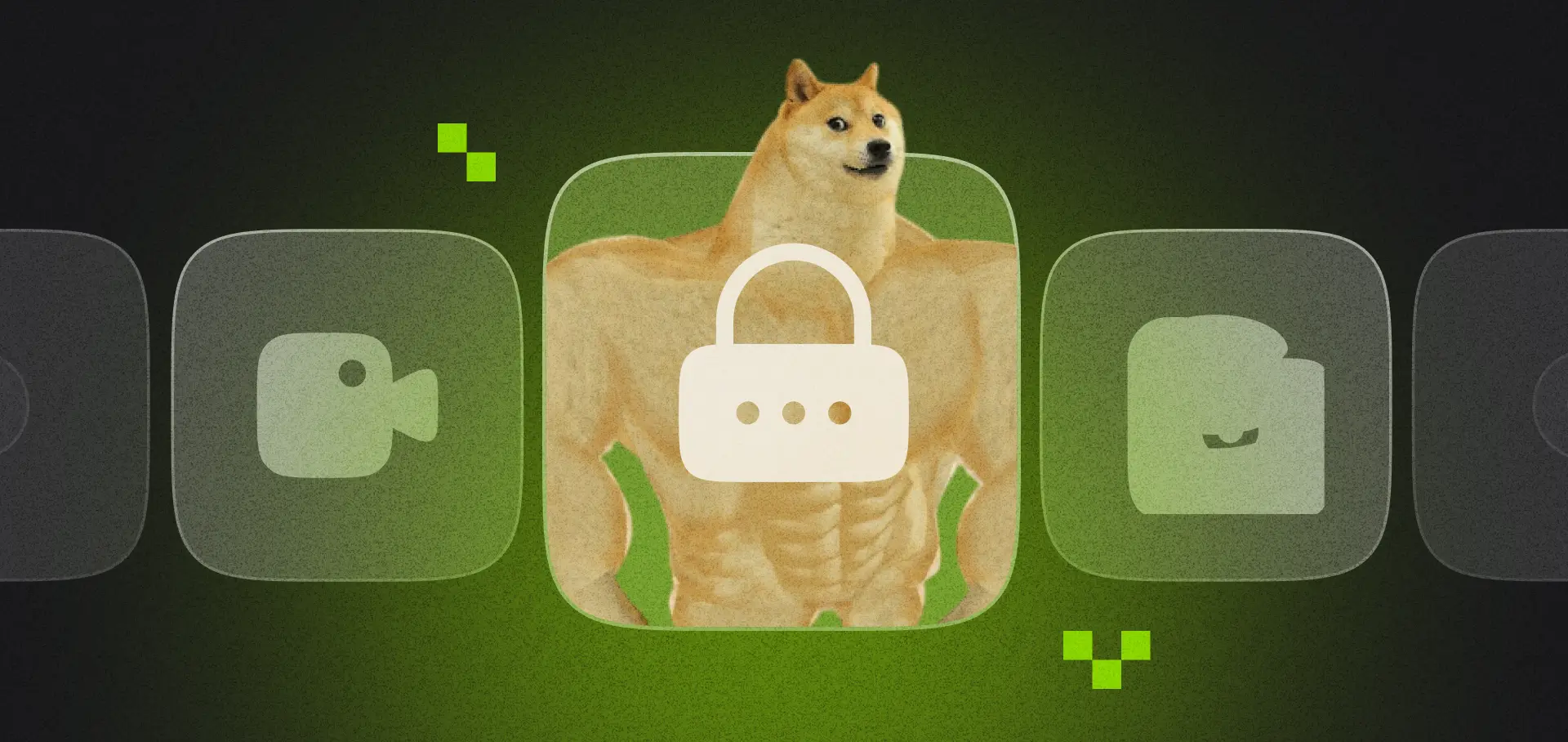
To develop a secure video communication app for Android, you'll need to implement several critical security measures. Start by integrating WebRTC for end-to-end encryption and OAuth 2.0 for user authentication. Include strong security features like the Signal Protocol for encrypted transmissions, role-based access control, and multi-factor authentication. You'll also need to guarantee GDPR compliance, implement secure transport protocols like SRTP, and establish regular security audits. Focus on balancing strong security with peak app performance through efficient code implementation and thorough testing protocols. The following sections will reveal the essential technical components for building your secure video application.
Key Takeaways
- Implement WebRTC with end-to-end encryption protocols to ensure secure real-time video communication between Android app users.
- Integrate OAuth 2.0 authentication and role-based access control to verify user identities and manage permissions effectively.
- Use secure transport protocols like SRTP combined with automatic key rotation mechanisms to protect video streams during transmission.
- Implement comprehensive user permissions management for camera, microphone, and storage access with explicit consent requirements.
- Establish regular security testing protocols, including vulnerability assessments and penetration testing of all API endpoints and connections.
💡 Ready to implement these security features in your video app?
Our team has 19+ years of experience in secure video development. Schedule a free consultation to discuss your requirements.
Understanding Secure Video Communication
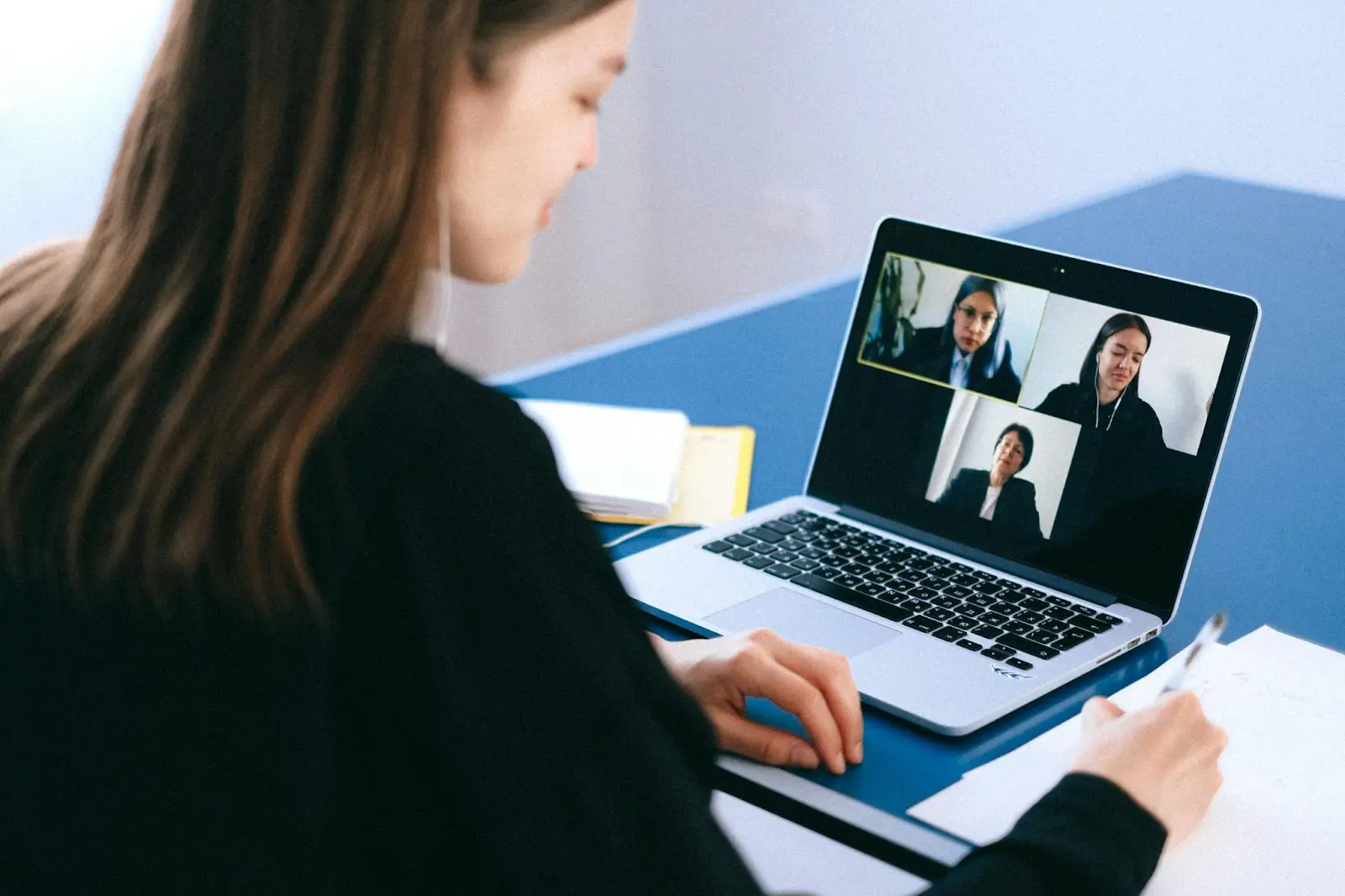
Your Android video communication app needs strong security features to protect users' private conversations and sensitive data from increasingly advanced cyber threats.
You'll face key technical challenges including end-to-end encryption implementation, secure key exchange protocols, and protection against man-in-the-middle attacks during video streaming.
Modern Android development frameworks offer built-in security solutions like the Android Keystore system, WebRTC's secure protocols, and encryption libraries that you can integrate to create a trusted video communication platform.
Why Secure Video Communication Matters Today
While video communication has become essential for business and personal connections, securing these digital interactions is now more critical than ever. Your apps need strong security features to protect users from data breaches and unauthorized access.
Modern secure video conferencing platforms implement advanced encryption standards and two-factor authentication to guarantee privacy. Leading platforms like Zoom and Secure Frame have demonstrated the essential role of end-to-end encryption protocols in safeguarding user privacy during online meetings (Park et al., 2023).
Key security measures you'll need to implement:
- End-to-end encryption for all video and audio streams
- Two-factor authentication integration with biometric options
- Real-time monitoring for suspicious activities and potential threats
- Secure data storage with encrypted backups
- Access control mechanisms with role-based permissions
Understanding these requirements helps you develop applications that meet current security standards while maintaining user privacy.
Implementing these features from the start prevents costly security retrofitting and builds user trust in your platform.
Key Security Challenges in Video Communication
Despite strong security measures being available, video communication apps face several critical security challenges that developers must address head-on.
When you're developing secure video conferencing software, you'll need to tackle unauthorized access attempts, data interception during transmission, and potential vulnerabilities in user authentication systems. Your primary security issues will include protecting against man-in-the-middle attacks and ensuring proper encryption at rest for stored data.
Implementing sturdy cybersecurity measures requires careful attention to both server-side and client-side security. You'll need to integrate multi-factor authentication to verify user identities and implement end-to-end encryption protocols to protect video streams. Furthermore, you must consider challenges like secure key exchange mechanisms, protecting metadata, and maintaining security without compromising the app's performance or user experience.
How Android Apps Address These Challenges
Android developers have strong tools at their disposal to combat video communication security threats. When you're implementing secure video conferencing solutions, it's vital to integrate multiple security layers that protect both your app and users' privacy.
Here are some essential strategies to consider when building a secure video communication app:
- Implement end-to-end encryption protocols like WebRTC for real-time communication on mobile devices.
- Create a user-friendly interface that displays security status and permissions.
- Integrate OAuth 2.0 and token-based authentication systems for secure user verification.
- Develop transparent privacy policies that comply with GDPR and other regulations.
- Employ secure APIs from major video conferencing solutions providers for proven security frameworks.
These security measures help guarantee your Android video communication app maintains high protection standards while delivering seamless functionality.
Organizations that conduct regular security audits are more likely to identify vulnerabilities before they can be exploited, significantly reducing the risk of data breaches (Ling, 2023). Regular security audits and updates keep your implementation current with evolving threats and industry best practices.
🔒 Looking for experts in secure video app development?
We've successfully delivered 100+ video streaming projects with advanced security features. Check out our portfolio or contact us to discuss your project.
Essential Security Components
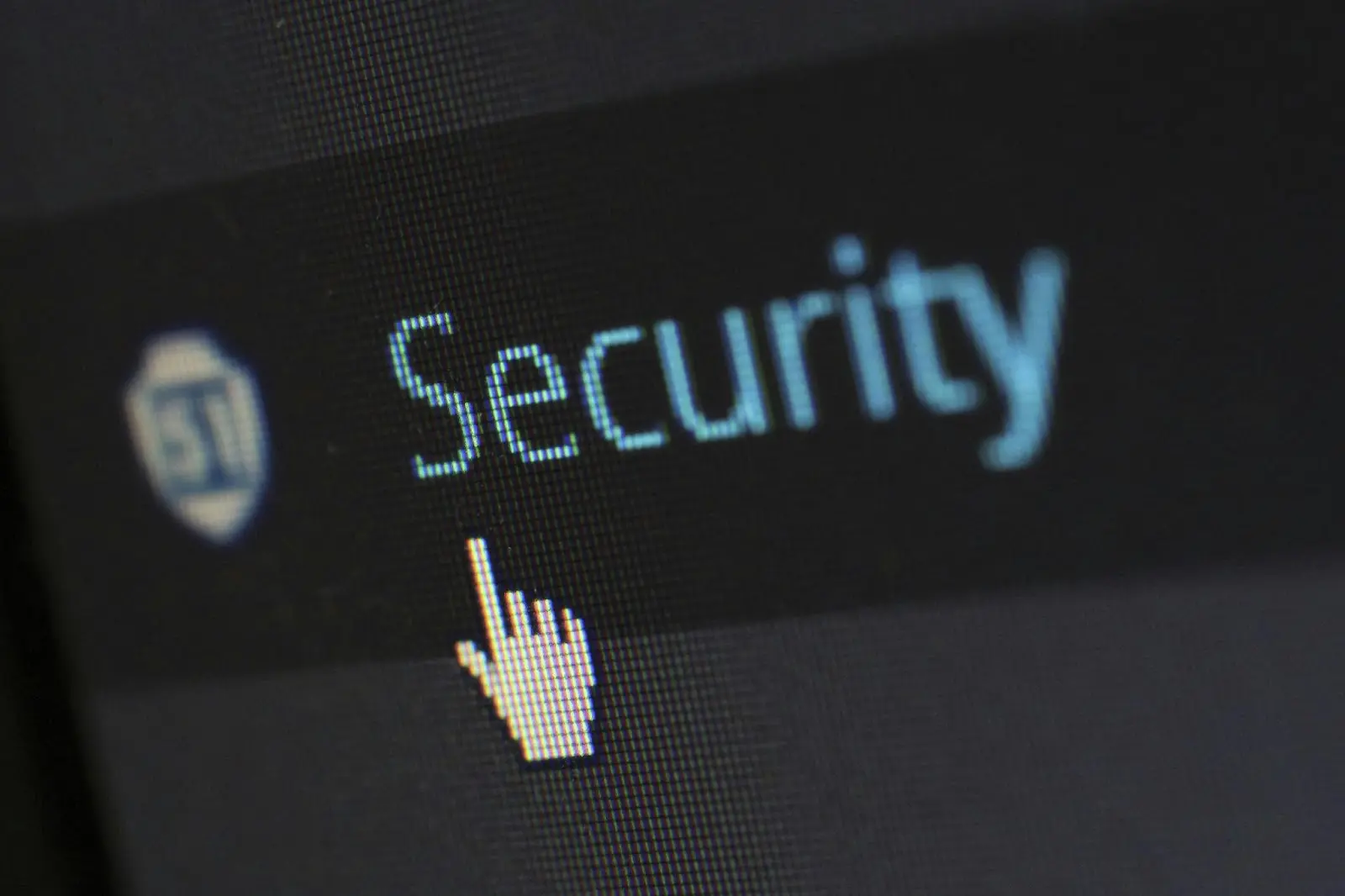
To build secure video communication in your Android app, you'll need to implement three critical security components: end-to-end encryption to protect video streams from unauthorized access, strong authentication mechanisms to verify user identities, and secure transport protocols like SRTP or WebRTC.
Your authentication system should incorporate multi-factor authentication options and role-based access control to manage user permissions effectively. Using industry-standard protocols such as TLS for signaling and DTLS-SRTP for media encryption will guarantee your video communications remain protected against common security threats.
End-To-End Encryption Implementation
When implementing video communication apps, end-to-end encryption serves as your first line of defense against data breaches and unauthorized access. This security measure has become increasingly standard in the industry, with approximately 70% of popular messaging applications and video conferencing tools now incorporating end-to-end encryption to enhance user privacy and security (Scheffler & Mayer, 2023).
To develop a secure video conferencing service, you'll need to integrate strong encryption protocols that address privacy risks and cybersecurity issues. Your secure solution should prioritize data protection at every transmission point.
Here are some key measures to consider:
- Implement the Signal Protocol for reliable end-to-end encryption of video streams
- Use WebRTC's built-in encryption capabilities combined with DTLS-SRTP
- Integrate Perfect Forward Secrecy to protect past sessions
- Set up automatic key rotation mechanisms to enhance security
- Enable certificate pinning to prevent man-in-the-middle attacks
During development, confirm your encryption implementation includes proper key management, secure key exchange mechanisms, and regular security audits to maintain the highest protection standards for your users' communications.
Authentication and Access Control Methods
Strong authentication and access control serve as critical pillars for any video communication app's security architecture.
You'll need to implement multiple authentication and access control methods to protect your users' video conferences. Start by integrating a reliable authenticator app that generates time-based one-time passwords for two-factor authentication. When designing secure video conference features, incorporate password-protected meetings with unique meeting IDs and waiting rooms. This lets hosts verify participants before granting access.
Consider implementing role-based access control to manage different user permission levels effectively. To maintain compliance with cyber security authority guidelines, regularly update your authentication protocols and monitor for potential vulnerabilities. You should also enforce strong password policies and implement automatic session timeouts to prevent unauthorized access to ongoing video calls.
Secure Transport Protocols for Video
Reliable video communication depends on implementing secure transport protocols that protect data during transmission. When developing video conferencing software, you'll need to prioritize online privacy and implement key security features to safeguard user data.
To ensure the security and integrity of real-time communication systems, consider implementing the following protocols and frameworks:
- Use SRTP (Secure Real-time Transport Protocol) to encrypt video and audio streams during transmission.
- Implement TLS (Transport Layer Security) for securing signaling data and authentication processes.
- Enable DTLS (Datagram Transport Layer Security) to protect peer-to-peer communications.
- Configure ICE (Interactive Connectivity Establishment) frameworks to handle NAT traversal securely.
- Employ STUN/TURN servers to aid in secure connection establishment.
Your threat protection strategy should include regular security audits, certificate validation, and proper implementation of these protocols.
Remember to keep transport security configurations updated to address emerging vulnerabilities and maintain compliance with industry standards.
Building the Secure Video App
To build a secure video communication app, you'll need to start with a properly configured Android development environment that includes the latest WebRTC libraries and security tools. Our experience developing ProVideoMeeting has shown that utilizing WebRTC alongside HTML5 ensures optimal performance and automatic quality adaptation based on connection stability.
You can strengthen your app's security by implementing end-to-end encryption using established cryptographic libraries like Signal Protocol or OpenSSL while ensuring proper certificate validation and secure key exchange mechanisms. For instance, in ProVideoMeeting, we implemented 12-AES encryption for the digital signature system, making it compliant with U.S. legal requirements and ensuring secure document handling during video conferences.
Managing user permissions and authentication requires implementing strong session handling, OAuth 2.0 integration, and runtime permission checks for camera and microphone access. Drawing from our ProVideoMeeting implementation, we enhanced security by incorporating additional verification methods such as SMS verification and snapshot authentication for identity confirmation, particularly crucial for legal document signing features.
Setting up the Development Environment
Setting up a secure video app development environment requires three essential components: Android Studio, the latest SDK tools, and appropriate emulators for testing.
You'll need to configure your workspace to support video conferencing tools and security features while maintaining an intuitive interface for unlimited meetings and screen-sharing capabilities.
Essential setup steps include:
- Installing Android Studio with the complete Android SDK package
- Configuring NDK for native code development and enhanced security
- Setting up WebRTC libraries for real-time communication
- Installing SSL certificates for encrypted data transmission
- Configuring Firebase for user authentication and cloud services
After installation, verify your development environment by running basic video communication tests on both physical devices and emulators.
This guarantees proper functionality across different Android versions and screen sizes before proceeding with advanced feature implementation.
Implementing WebRTC Security Features
Building secure WebRTC features starts with implementing end-to-end encryption protocols in your Android video app.
You'll need to configure DTLS-SRTP encryption to protect real-time media streams and establish secure peer connections that meet industry security standards. To prevent unauthorized access, implement user authentication through secure tokens and session management. A comparative study found that token-based authentication systems significantly reduce unauthorized access incidents compared to traditional username and password methods (Pratama et al., 2018).
You can enhance basic security by adding certificate pinning and enabling perfect forward secrecy in your video conferencing apps.
While absolute privacy isn't guaranteed, you should encrypt all signaling data using TLS 1.3 or higher. Don't forget to validate incoming connections, implement proper key exchange mechanisms, and regularly update your security certificates.
Consider adding features like secure room codes, waiting rooms, and host controls to provide additional layers of protection for your users' communication sessions.
Integrating Encryption Libraries
When securing your Android video app, popular encryption libraries like Signal Protocol, OpenSSL, and Google Tink provide essential building blocks for strong data protection.
A survey of developers revealed that 65% of mobile app developers prioritize established encryption libraries like OpenSSL and Google Tink to secure user data in their applications (Alammary et al., 2022). These libraries offer decent security features that you'll need to integrate into your video conferencing platform for reliable protection of user data and communications.
Below are essential steps to secure user data and communication security:
- Implement double encryption for all video calling functionality using both in-transit and at-rest encryption methods.
- Set up secure storage for user credentials and session keys using Android Keystore.
- Configure end-to-end encryption for real-time video streams.
- Enable perfect forward secrecy to protect past communications.
- Implement certificate pinning to prevent man-in-the-middle attacks.
Remember to regularly update your encryption libraries to maintain security standards and protect against newly identified vulnerabilities.
Testing your implementation thoroughly helps guarantee proper encryption integration across all app components.
Managing User Permissions and Sessions
Sturdy user permissions and session management form the foundation of a secure Android video communication app.
You'll need to implement thorough user permissions that protect sensitive data while enabling unlimited video-calling functionality. Configure your app to request explicit consent for camera, microphone, and storage access during the initial setup. Integrate a secure browser component for web-based features and guarantee your privacy notice clearly outlines data handling practices.
When designing meeting controls, implement role-based permissions that define what actions different user types can perform during video sessions. Set up automatic session timeouts and implement secure token-based authentication to prevent unauthorized access.
Store session data in encrypted formats and establish protocols for graceful session termination when users exit the app or lose connectivity.
Advanced Security Implementation
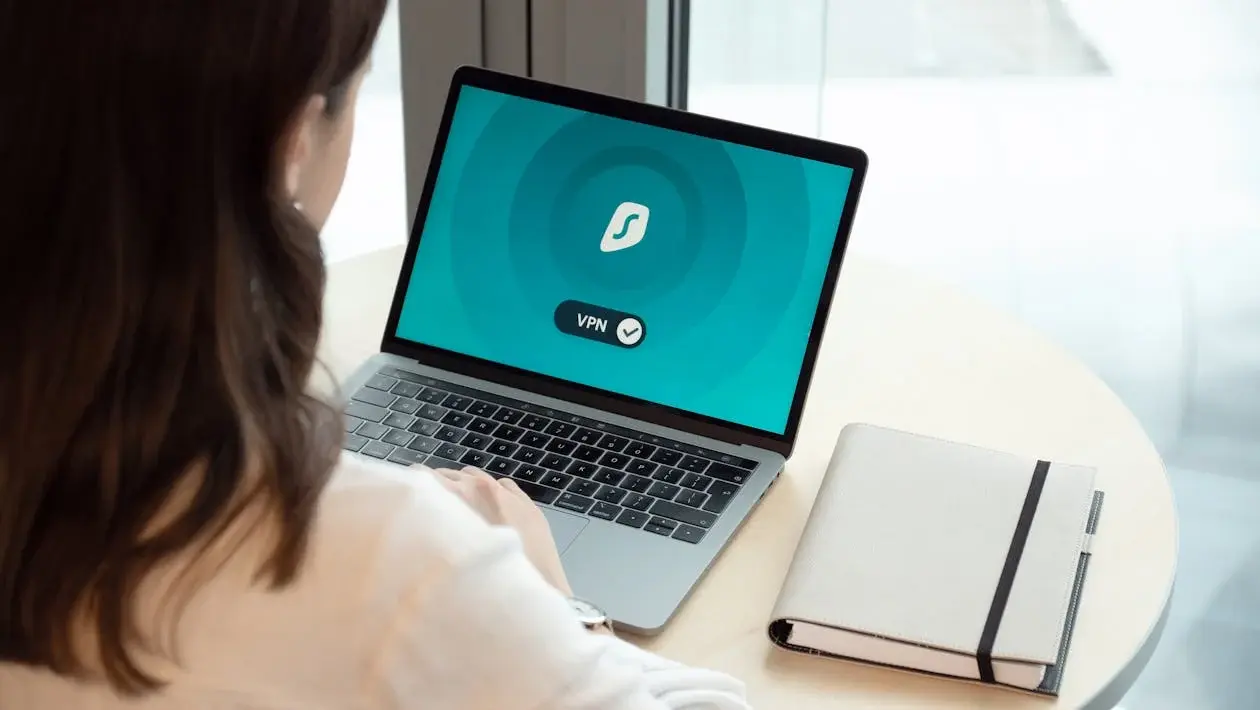
To enhance your video communication app's security, you'll need to implement real-time threat detection systems that can identify and block potential attacks while users are streaming.
You can strengthen data protection by ensuring compliance with GDPR and HIPAA regulations through encrypted data storage, user consent management, and proper documentation of data handling procedures. Your security features should be optimized for performance by using efficient encryption algorithms, implementing smart caching mechanisms, and minimizing the impact on video streaming latency.
Real-Time Threat Detection Systems
Since video communication apps handle sensitive data streams, implementing real-time threat detection systems is crucial for protecting users from malicious attacks and unauthorized access. You'll need to integrate monitoring tools that continuously scan for potential security breaches across your video conferencing service provider and cloud storage providers.
Here's a list of key actions you can implement to safeguard your systems:
- Implement automated anomaly detection to identify unusual patterns in user behavior.
- Deploy AI-powered scanning systems to monitor encrypted business communications.
- Set up real-time alerts for suspicious activities like unauthorized access attempts.
- Configure automated response protocols to isolate potential threats instantly
- Establish continuous monitoring of network traffic patterns for data leakage.
These systems should work seamlessly in the background, analyzing traffic patterns, user behaviors, and data transfers while maintaining peak app performance and user privacy.
Regular updates to threat detection algorithms guarantee protection against emerging security risks.
Compliance With GDPR and HIPAA
Regulatory compliance represents a critical foundation for video communication apps handling sensitive user data across healthcare and international markets.
When developing your Android app, you'll need to implement protection regulations like GDPR for European users and HIPAA for healthcare applications. These standards require specific security features, including end-to-end encryption for video conferences and strict cloud storage limits. Ensure your app includes clear user consent mechanisms, data retention policies, and documentation of security measures.
For remote team collaboration in healthcare settings, you'll need audit trails of all video sessions and secure methods for sharing patient information. Implement data minimization practices, collecting only essential information, and provide users with options to export or delete their data.
Regular security audits and updates will help maintain compliance with evolving regulations.
Performance Optimization for Security Features
Strong security features often compete with app performance, requiring careful optimization to maintain smooth video communication. When implementing security features in your video solutions, it's essential to balance protection with performance to guarantee a seamless user experience.
To achieve this balance, consider the following key strategies that can optimize both security and performance in your video solutions:
- Use asynchronous encryption methods to minimize impact on video streaming latency
- Implement custom developer integrations that utilize hardware acceleration for encryption processes
- Optimize unlimited cloud recordings by compressing data before applying security layers
- Configure security features to run on separate processing threads, preventing main UI slowdown
- Cache security certificates and tokens locally to reduce authentication overhead
Focus on performance optimization by monitoring key metrics like CPU usage, memory consumption, and battery drain.
Regular testing with different device configurations will help identify bottlenecks and confirm your security implementations don't compromise the app's responsiveness.
⚡ Want to ensure both security and performance in your video app?
Our team specializes in optimizing video streaming applications while maintaining robust security. Let's discuss your requirements - book a free 30-minute consultation.
Testing and Deployment
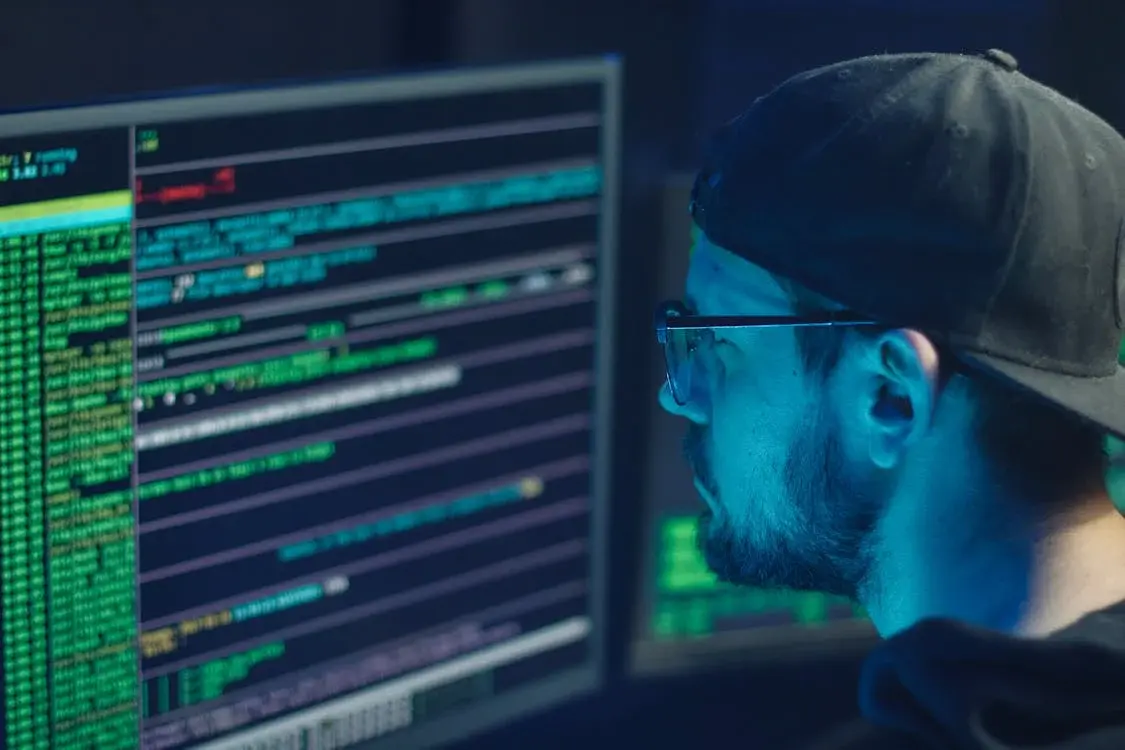
To guarantee your video communication app's security and performance, you'll need to implement extensive testing protocols that cover both security vulnerabilities and user experience metrics.
You can use Android's built-in testing frameworks alongside specialized security testing tools to verify encryption protocols, data handling, and network security features.
Before deployment, it's crucial to run thorough compatibility tests across different Android versions and devices while establishing a systematic approach for rolling out regular security updates and patches.
Security Testing Protocols
Security testing protocols serve as your first line of defense when developing video communication apps for Android.
When you're building apps in the video conferencing space, it's vital to implement thorough security measures that protect your users' data and communications, especially for features like unlimited meeting duration and screen share functionality.
Below are some key considerations for securing your app while maintaining an optimal experience for your users:
- Implement end-to-end encryption testing for all video and audio streams
- Conduct regular vulnerability assessments focusing on authentication mechanisms
- Test session management security to prevent unauthorized access
- Verify data privacy compliance across your range of functionality
- Perform penetration testing on all API endpoints and server connections
These protocols guarantee your app meets industry security standards while maintaining performance.
Remember to document all security test results and maintain an ongoing testing schedule as you update your application's features and capabilities.
Performance and Compatibility Testing
Reliable performance and compatibility testing form the foundation of successful Android video communication apps.
You'll need to evaluate your app's behavior across different network conditions, device types, and Android versions to guarantee consistent quality during online meetings. Begin by testing core functionalities with various service providers and network speeds. Monitor CPU usage, memory consumption, and battery drain while running your app's full range of features.
Your testing protocol should include scenarios that match your target pricing plan requirements, particularly if you're developing a solution for enterprises. Implement automated testing frameworks to verify compatibility across multiple Android versions and screen sizes. Use Android's built-in testing tools like Espresso for UI testing and JUnit for unit tests.
Document performance benchmarks and establish minimum device requirements to maintain quality standards.
Deployment Security Checklist
A thorough deployment security checklist works alongside performance testing to protect your video communication app and its users. When developing mobile apps with video functionality, you'll need to guarantee extensive security measures are in place before deployment.
To ensure a comprehensive security approach, it's essential to address the following key areas before deploying your video communication app:
- Implement end-to-end encryption for all video streams and verify that personalization options don't compromise security protocols.
- Audit screen annotation features to prevent potential data leakage or unauthorized access.
- Configure secure authentication methods and validate subscription model payment gateways.
- Test for vulnerabilities in file sharing and chat features, guaranteeing proper encryption at rest.
- Review permissions and access controls, particularly for camera and microphone usage.
Complete each security checklist item methodically, documenting any issues found and their resolutions. This systematic approach helps maintain user trust and protects sensitive data while supporting your app's core video communication features.
Maintaining Security Updates
While developing video communication apps requires strong initial security measures, maintaining consistent security updates through systematic testing and deployment is equally essential. During our development of Speed Space for Revo Studio, we learned firsthand how critical this is for professional video production platforms where intellectual property protection is paramount.
You'll need to establish a regular schedule for security updates to protect your solid video conferencing solution against emerging threats. In Speed Space's role-based access control system, we implement regular security audits to ensure Admin and Production Member privileges remain properly segregated and protected.
For larger organizations, implement automated testing pipelines that verify security patches don't disrupt existing virtual meetings functionality. This became particularly crucial for Speed Space's real-time production management features, where even minor disruptions could affect live recording sessions and stream quality.
Monitor your productivity tools for vulnerabilities using automated scanning systems, and prioritize critical security updates based on risk assessment. In our experience with Speed Space's cloud-based post-production workflow, we found this especially important for protecting sensitive recorded content and maintaining client confidentiality.
Deploy updates incrementally to detect potential issues before full rollout, and maintain detailed documentation of all security changes. This approach proved invaluable when updating Speed Space's studio and set management features, ensuring that production teams could continue working without interruption.
Set up automated alerts for new security vulnerabilities in third-party dependencies, and guarantee your team can quickly respond to critical patches when needed. This is particularly vital for platforms like Speed Space that integrate multiple features such as video conferencing, real-time production tools, and cloud storage capabilities.
Our Expertise in Secure Video Communication Development
Since 2005, we've specialized exclusively in video streaming and real-time software development, making us pioneers in secure video communication solutions. Our journey parallels the evolution of video technology itself - from the early days of WebRTC to today's advanced encryption standards. With over 625 successfully completed projects and a perfect 100% success rate on Upwork, we've consistently delivered secure video solutions across various sectors, including telemedicine platforms handling sensitive patient data and educational systems serving thousands of users daily.
We have a deep specialization in video security implementation. Every developer on our team undergoes a rigorous selection process (only 1 in 50 candidates makes the cut) and must complete a mandatory 2-week video security project before working on client applications. This specialized focus has led to significant achievements, such as developing BrainCert - the world's first HTML5+WebRTC learning management system, which now generates $10M in annual revenue and serves as a testament to our ability to build secure, scalable video solutions.
Our expertise particularly shines in implementing complex security features while maintaining optimal performance. Through our partnerships with leading WebRTC solution providers and network video solutions experts, we gain early access to cutting-edge security technologies and protocols. This privileged position allows us to implement the most current security measures in our clients' applications, from advanced encryption protocols to sophisticated authentication systems.
Whether you're building a telemedicine platform requiring HIPAA compliance or a corporate video conferencing solution needing enterprise-grade security, our team's specialized knowledge ensures your application meets the highest security standards while delivering seamless performance.
Frequently Asked Questions
How Can Low-Bandwidth Users Maintain Video Quality Without Compromising Security?
You'll need to implement flexible bitrate streaming, utilize efficient codecs like H.264/H.265, enable dynamic resolution scaling, and leverage WebRTC's bandwidth estimation features while maintaining end-to-end encryption for security.
What Strategies Exist for Handling Video Communication During Intermittent Network Switches?
You'll want to implement flexible bitrate streaming, buffer pre-loading, and WebRTC's ICE protocol. Use seamless connection handoffs, maintain session tokens, and cache video frames for quick recovery during network changes.
Can Existing Video Codecs Be Modified to Enhance Encryption Performance?
You can optimize encryption by customizing VP8/VP9 or H.264 codecs' parameters, implementing hardware acceleration, or using selective encryption methods to only encrypt critical frame data, improving overall performance while maintaining security.
How Do Different Android Versions Affect Secure Video Implementation Across Devices?
You'll need to handle API level differences, as newer Android versions support enhanced codec security features. Guarantee backward compatibility by implementing fallback options and version-specific code paths for older devices.
What Are the Battery Optimization Techniques for Continuous Secure Video Streaming?
You'll improve battery life by implementing frame rate throttling, using hardware acceleration, compressing video streams, enabling background optimization flags, and initializing wake locks only during active streaming sessions.
To Sum Up
You've now got the essential tools to develop a secure Android video communication app. By implementing end-to-end encryption, strong authentication, and WebRTC protocols, you'll protect your users' privacy while delivering smooth video calls. Remember to regularly test your security measures and stay updated with Android's latest security patches. With these fundamentals in place, you're ready to deploy a trustworthy video communication solution.
🚀 Ready to build your secure video communication app?
With our proven expertise in AI integration and video streaming, we can help you create a robust, secure solution. View our AI development services or schedule a call to get started.
References:
Alammary, A., Alshaikh, M., & Pratama, A. (2022). Awareness of security and privacy settings in video conferencing apps among faculty during the COVID-19 pandemic. Peerj Computer Science, 8, e1021. https://doi.org/10.7717/peerj-cs.1021
Ling, M. (2023). Machine-learning-based network sparsification modeling for IoTs security analysis. Proceedings Volume 12748, 5th International Conference on Information Science, Electrical, and Automation Engineering (ISEAE 2023), 188. https://doi.org/10.1117/12.2690061
Park, Y., Yoo, H., Ryu, J., et al. (2023). End-to-End PQC Encryption Protocol for GPKI-based Video Conferencing System. Appl. Syst. Innov, 6, 66. https://doi.org/10.20944/preprints202305.2077.v1
Pratama, I., Linawati, L., & Sastra, N. (2018). Token-based Single Sign-on with JWT as Information System Dashboard for Government. Telkomnika (Telecommunication Computing Electronics and Control), 16(4), 1745. https://doi.org/10.12928/telkomnika.v16i4.8388
Scheffler, S. and Mayer, J. (2023). SoK: Content Moderation for End-to-End Encryption. Proceedings on Privacy Enhancing Technologies, 2023(2), pp. 403-429. https://doi.org/10.56553/popets-2023-0060
Comments